1. Introduction
In this article, we learn about SQL injection security vulnerability in web application. We see an example of SQL Injection, learn in in-depth how it works, and see how we can fix this vulnerability. We use PHP and MySQL for the examples. The SQL injection is the top exploit used by hackers and is one of the top attacks enlisted by the OWASP community.
2. What is SQL Injection
SQL Injection is a attack mostly performed on web applications. In SQL Injection, attacker injects portion of malicious SQL through some input interfaces like web forms. These injected statements goes to the database server behind a web application and may do unwanted actions like providing access to unauthorised person or deleting or reading sensitive information.
The SQL Injection vulnerability may affect any application powered by database supporting SQL like Oracle, MySQL and others.
SQL Injection attacks are one of the widest used, oldest, and very dangerous application vulnerabilities. The OWASP organization (Open Web Application Security Project) lists SQL Injections in their OWASP Top 10 document as the top threat to web application security.
3. Example of SQL Injection
Let’s create a form in HTML:
- <!DOCTYPE html>
- <html>
- <body>
- <h2>SQL injection in web applications</h2>
- <form action="/form-handler.php">
- Username:<br>
- <input type="text" name="username" value="">
- <br>
- Password:<br>
- <input type="password" name="password" value="">
- <br><br>
- <input type="submit" value="Submit">
- </form>
- </body>
- </html>
When we click on submit, the form above submits to below PHP script:
- <?php
- mysql_connect('localhost', 'root', 'root');
- mysql_select_db('bootsity');
- $username = $_POST["username"];
- $password = $_POST["password"];
- $query = "SELECT * FROM Users WHERE username = " . $username . " AND password =" . $password;
- $re = mysql_query($query);
- if (mysql_num_rows($re) == 0) {
- echo 'Not Logged In';
- } else {
- echo 'Logged In';
- }
- ?>
4. How SQL Injection works
In the above example, assume that the user fills up the form as below:
- Username: ' or '1'='1
- Password: ' or '1'='1
Now our $query becomes:
SELECT * FROM Users WHERE username='' or '1'='1' AND password='' or '1'='1';
This query always returns some rows and results in printing Logged In on the browser. So, here the attacker doesn’t know any username or password that are register in the database, but the attacker is still able to log in.
5. Fixing SQL Injection
Now we understand how SQL injection works in PHP. Generally, the best solution is to use prepared statements and parameterized queries. When we use prepared statements and parameterized queries, the SQL statements are parsed separately by the database engine. Let us see these approaches below:
5.1 Using PDO
We can change our form-handler.php to use PDO:
- <?php
- $dsn = "mysql:host=localhost;dbname=bootsity";
- $user = "root";
- $passwd = "root";
- $pdo = new PDO($dsn, $user, $passwd);
- $username = $_POST["username"];
- $password = $_POST["password"];
- $stmt = $pdo->prepare('SELECT * FROM Users WHERE username = :username AND password = :password');
- $stmt->bindParam(':username', $username);
- $stmt->bindParam(':password', $password);
- $stmt->execute();
- if (count($stmt) == 0) {
- echo 'Not Logged In';
- } else {
- echo 'Logged In';
- }
- https://article-realm.com/article/Reference-Education/Philosophy/2509-What-is-SQL-Injection-and-how-to-fix-it.html
URL
https://bootsity.com/php/what-is-sql-injection-and-how-to-fix-itTutorial article to describe SQL Injection vulnerability in web application with example in PHP and MySQL
Reviews
Comments
Most Recent Articles
- Apr 21, 2022 Schnell Englisch lernen für Anfänger, Fortgeschrittene & Business by englisch lernen
- Jan 28, 2022 Wie man einen hochwertigen muttersprachlichen Englischlehrer online findet-einige Tipps by Alles Englisch
- Dec 29, 2021 In China, Mandarin is the Sole Official Language by Chinesegalaxy Kids
- Dec 3, 2021 Download the Best App for Learning Chinese by Chinesegalaxy Kids
- Aug 1, 2021 Discover an Easier Scope to Improve Your Conversation Skill in English by Mark Sheldon
Most Viewed Articles
- 927 hits Follow Top 6 Ways to Improve Communication Skills by Patricia Grays
- 391 hits Get Familiar with an Amazing Way to Improve Your English Communication by Mark Sheldon
- 383 hits Java Training Course In Noida by Digitaledge
- 346 hits Discover an Easier Scope to Improve Your Conversation Skill in English by Mark Sheldon
- 333 hits O Level Chinese - A way to learn Chinese making use of modern technical developments by Words Kitchen
Popular Articles
In today’s competitive world, one must be knowledgeable about the latest online business that works effectively through seo services....
77367 Views
Are you caught in between seo companies introduced by a friend, researched by you, or advertised by a particular site? If that is the...
32685 Views
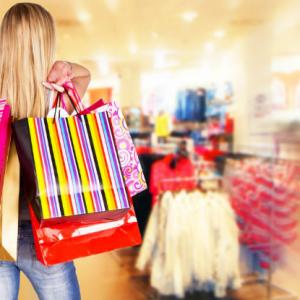
Walmart is being sued by a customer alleging racial discrimination. The customer who has filed a lawsuit against the retailer claims that it...
13703 Views
If you have an idea for a new product, you can start by performing a patent search. This will help you decide whether your idea could become the...
11085 Views
Statistics
Members | |
---|---|
Members: | 15316 |
Publishing | |
---|---|
Articles: | 63,054 |
Categories: | 202 |
Online | |
---|---|
Active Users: | 877 |
Members: | 16 |
Guests: | 861 |
Bots: | 12337 |
Visits last 24h (live): | 2062 |
Visits last 24h (bots): | 25588 |