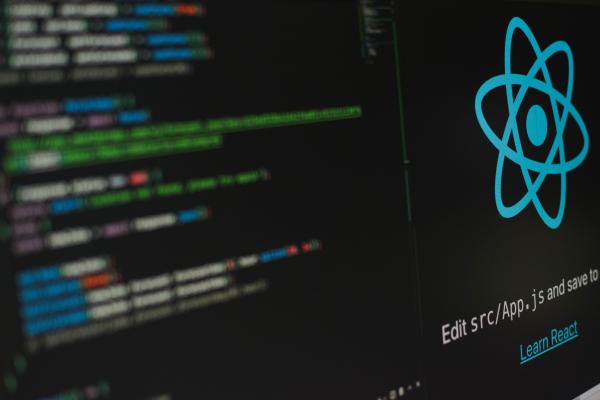
Real-time chat applications are an essential tool for communication and collaboration in today's digital age. Whether you're working on a team project, catching up with friends and family, or just looking for a way to stay connected, real-time chat can be a quick and convenient way to exchange messages and ideas.
In this article, we'll explore how to build a real-time chat application using two popular technologies: ReactJS and Firebase. ReactJS is a powerful front-end framework for building user interfaces, while Firebase is a real-time database and backend-as-a-service platform that offers authentication, storage, and more. Together, these technologies provide a powerful and flexible toolset for building real-time chat applications that can scale to meet the needs of any user base.
By following the steps outlined in this article, you'll learn how to set up a new ReactJS project with Firebase, design a chat interface, build real-time chat functionality, and implement user authentication. Along the way, we'll cover best practices for design and development, so you can create a chat application that is both functional and user-friendly.
So if you're ready to dive into the world of real-time chat application development, let's get started!
Setting up the environment
ReactJS is a popular and powerful front-end framework for building user interfaces, while Firebase is a real-time database and backend-as-a-service platform that offers authentication, storage, and more. When combined, they provide a comprehensive toolset for building real-time chat applications.
In this section, we will go through the process of setting up a new ReactJS project with Firebase, step-by-step.
-
Create a new Firebase project
The first step is to create a new Firebase project. To do this, go to the Firebase console (https://console.firebase.google.com/) and click on "Add Project". Give your project a name and select your preferred location.
-
Set up authentication
To enable authentication for your chat application, you'll need to set up Firebase Authentication. Go to the Firebase console and click on "Authentication" in the left-hand menu. Then, click on "Set up sign-in method" and select the authentication providers you want to use (such as Google, Facebook, or email/password).
-
Configure Firebase SDK in your ReactJS project
To use Firebase in your ReactJS project, you'll need to install the Firebase SDK and configure it. Here's how:
-
In your terminal, navigate to the root directory of your ReactJS project.
-
Run the following command to install the Firebase SDK:
npm install firebase
-
Once the SDK is installed, you'll need to initialize it with your Firebase project credentials. To do this, create a new file called firebase.js in the src directory of your project.
-
In firebase.js, import the Firebase SDK and initialize it with your Firebase project credentials:
import firebase from "firebase/app";
import "firebase/auth";
import "firebase/database";
const firebaseConfig = {
// Your Firebase project config goes here
};
firebase.initializeApp(firebaseConfig);
export default firebase;
-
Finally, import the firebase.js module in your ReactJS components where you want to use Firebase, like this:
import firebase from "./firebase";
Now that you have set up your environment, you're ready to start building your real-time chat application
Designing the chat interface
In a real-time chat application, the interface plays a crucial role in making the user experience smooth and enjoyable. A poorly designed interface can lead to confusion and frustration, while a well-designed interface can help users navigate the application and communicate effectively with others.
Here are some principles to keep in mind when designing the chat interface:
-
Keep it simple: A simple and clean interface can help users focus on the conversation and avoid distractions.
-
Make it intuitive: The interface should be easy to use and navigate, with clear labels and instructions.
-
Provide feedback: Users should receive feedback when they perform an action, such as sending a message or joining a chat.
-
Make it customizable: Allow users to customize the interface to suit their preferences, such as choosing a theme or changing the font size.
With these principles in mind, we'll now use ReactJS to build a basic chat interface. We'll create a component called Chat that will contain a message input field, a message display area, and a list of online users.
Here's the code for the Chat component:
import React, { useState } from "react";
function Chat() {
const [currentUser, setCurrentUser] = useState(null);
const [messages, setMessages] = useState([]);
const [onlineUsers, setOnlineUsers] = useState([]);
return (
<div className="chat">
<div className="users">
<h2>Online Users:</h2>
<ul>
{onlineUsers.map((user) => (
<li key={user.uid}>{user.displayName}</li>
))}
</ul>
</div>
<div className="messages">
<ul>
{messages.map((message) => (
<li key={message.timestamp}>
<span className="user">{message.displayName}</span>
<span className="message">{message.text}</span>
</li>
))}
</ul>
<form>
<input type="text" placeholder="Type your message here" />
<button type="submit">Send</button>
</form>
</div>
</div>
);
}
export default Chat;
In this code, we're using the useState hook to create three state variables: currentUser, messages, and onlineUsers. We're rendering these variables in the JSX, with the onlineUsers list on the left and the messages list on the right.
We've also added a message input field and a button that will allow the user to send a message. However, at this point, the input field and button are not yet functional. We'll add the necessary code to handle sending messages in the next section.
Building real-time chat functionality
Real-time chat is a communication method that enables users to send and receive messages instantly, without delay. In a chat application, real-time functionality is critical for ensuring that messages are delivered promptly and that users can respond quickly to incoming messages.
In our chat application, we'll use Firebase's real-time database to build real-time chat functionality. When a user sends a message, the message will be added to the database, and all other users in the chat will receive the message in real-time. Similarly, when a user joins or leaves the chat, the list of online users will be updated in real-time for all other users.
Here's the code for updating the messages and onlineUsers state variables in real-time:
function Chat() {
// ...
useEffect(() => {
firebase
.database()
.ref("messages")
.on("value", (snapshot) => {
const messages = [];
snapshot.forEach((childSnapshot) => {
const message = childSnapshot.val();
messages.push(message);
});
setMessages(messages);
});
firebase
.database()
.ref(".info/connected")
.on("value", (snapshot) => {
if (snapshot.val() === true) {
const userRef = firebase.database().ref(`users/${currentUser.uid}`);
userRef.onDisconnect().remove();
userRef.set({
uid: currentUser.uid,
displayName: currentUser.displayName,
});
}
});
firebase
.database()
.ref("users")
.on("value", (snapshot) => {
const users = [];
snapshot.forEach((childSnapshot) => {
const user = childSnapshot.val();
users.push(user);
});
setOnlineUsers(users);
});
}, [currentUser]);
function handleSendMessage(event) {
event.preventDefault();
const text = event.target.elements.message.value;
if (text.trim() !== "") {
const message = {
uid: currentUser.uid,
displayName: currentUser.displayName,
text: text,
timestamp: Date.now(),
};
firebase.database().ref("messages").push(message);
event.target.reset();
}
}
// ...
return (
<div className="chat">
<div className="users">
<h2>Online Users:</h2>
<ul>
{onlineUsers.map((user) => (
<li key={user.uid}>{user.displayName}</li>
))}
</ul>
</div>
<div className="messages">
<ul>
{messages.map((message) => (
<li key={message.timestamp}>
<span className="user">{message.displayName}</span>
<span className="message">{message.text}</span>
</li>
))}
</ul>
<form onSubmit={handleSendMessage}>
<input type="text" name="message" placeholder="Type your message here" />
<button type="submit">Send</button>
</form>
</div>
</div>
);
}
In this code, we're using Firebase's real-time database to listen for changes to the messages and users nodes. When a message is added to the database, we're updating the messages state variable to include the new message. Similarly, when a user joins or leaves the chat, we're updating the onlineUsers state variable to reflect the current list of online users.
We've also added a function called handleSendMessage that is called when the user submits a new message. This function retrieves the text from the message input field, creates a new message object, and adds it to the messages node in the database. Once the message is added to the database, all other users in the chat will receive the message in real-time and the messages state variable will be updated to reflect the new message.
Overall, this code provides the real-time chat functionality that we need to build a functional chat application. By using Firebase's real-time database, we're able to ensure that messages are delivered promptly and that the list of online users is always up-to-date.
Implementing user authentication
User authentication is an essential feature of any chat application as it ensures that only authorized users can access and participate in the chat. Firebase offers several authentication options, including email and password authentication, Google sign-in, and phone number authentication, among others. In this section, we will implement user authentication using email and password authentication.
To add user authentication to our chat application, we first need to enable email and password authentication in our Firebase project. To do this, we can navigate to the Firebase Console, select our project, and then select "Authentication" from the left-hand menu. From there, we can choose the "Sign-in method" tab and enable email and password authentication.
With email and password authentication enabled, we can now add the authentication functionality to our ReactJS chat application. We'll begin by importing the Firebase authentication module and creating state variables for the email, password, and current user. We'll also create two functions for handling login and logout, respectively. Here's what the code for this might look like:
import { auth } from './firebase';
import { useState, useEffect } from 'react';
function Chat() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [currentUser, setCurrentUser] = useState(null);
useEffect(() => {
const unsubscribe = auth.onAuthStateChanged(user => {
if (user) {
setCurrentUser(user);
} else {
setCurrentUser(null);
}
});
return unsubscribe;
}, []);
function handleLogin(e) {
e.preventDefault();
auth.signInWithEmailAndPassword(email, password)
.then(() => {
setEmail('');
setPassword('');
})
.catch(error => alert(error.message));
}
function handleLogout() {
auth.signOut();
}
// Rest of the chat functionality goes here
return (
// Chat interface JSX
);
}
export default Chat;
In this code, we're using the useEffect hook to listen for changes in the authentication state and update the currentUser state variable accordingly. We're also creating functions for handling login and logout using auth.signInWithEmailAndPassword and auth.signOut, respectively.
With the authentication functionality in place, we can now add a login form to our chat interface and update the chat functionality to only be available to authenticated users. Additionally, we can add a logout button to the chat interface that triggers the handleLogout function when clicked.
In summary, Firebase's authentication features provide a convenient and secure way to add user authentication to our ReactJS chat application. With email and password authentication enabled, we can create login and logout functionality that restricts chat access to authorized users.
Conclusion
In conclusion, building a real-time chat application with ReactJS and Firebase is a straightforward process that leverages the power of Firebase's real-time database and authentication features. By using ReactJS for the front-end interface and Firebase for the back-end database and authentication, we're able to create a fully functional chat application that updates in real-time and provides a secure and scalable user authentication system.
Throughout this article, we've covered the key steps involved in building a real-time chat application, from setting up the environment and designing the chat interface, to adding real-time chat functionality and implementing user authentication. By following these steps, you can create your own real-time chat application using the powerful combination of ReactJS and Firebase.
If you're looking to build a real-time chat application but don't have the in-house expertise to do so, you can hire ReactJS developer who are well-versed in Firebase and other technologies. With the right team in place, you can build a chat application that meets your unique needs and delivers the functionality and user experience that your users demand.
Article source: https://article-realm.com/article/Health-Fitness/37881-Building-a-Real-Time-Chat-Application-with-ReactJS-and-Firebase-A-Step-by-Step-Guide.html
Reviews
Comments
Most Recent Articles
- Nov 5, 2024 Clear Aligners and Digital Workflow: Streamlining Processes for a Seamless Treatment Experience by rajesh panii
- Oct 28, 2024 Book Budget Friendly and Safest Medical Transportation with Falcon Train Ambulance in Patna by Falcon Emergency
- Oct 28, 2024 Logra una Vida Sana con V-Zana: Tu Mejor Versión al Alcance by merleshay
- Oct 27, 2024 Non-Emergency Wheelchair Transportation - Bridging Mobility Gaps by Rosario Berry
- Oct 27, 2024 Non-Emergency Transportation Services - A Vital Lifeline for Communities by Rosario Berry
Most Viewed Articles
- 33028 hits Familiarize The Process Of SEO by Winalyn Gaspelos
- 2430 hits Very Important Ergonomic Office Furniture Brand You Should Know About by neck
- 2383 hits Get Solution of Hp Printer Offline Errors on Windows and Mac by shubhi gupta
- 2282 hits Cheap Domain Registration and Web Hosting in Nepal: AGM Web Hosting by Hari Bashyal
- 2276 hits Reasons Developers Should Switch to HTML5 ASAP by Guest
Popular Articles
In today’s competitive world, one must be knowledgeable about the latest online business that works effectively through seo services....
77514 Views
Are you caught in between seo companies introduced by a friend, researched by you, or advertised by a particular site? If that is the...
33028 Views
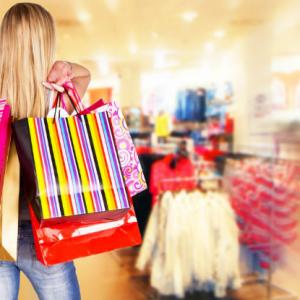
Walmart is being sued by a customer alleging racial discrimination. The customer who has filed a lawsuit against the retailer claims that it...
14055 Views
If you have an idea for a new product, you can start by performing a patent search. This will help you decide whether your idea could become the...
11257 Views
Statistics
Members | |
---|---|
Members: | 15673 |
Publishing | |
---|---|
Articles: | 64,357 |
Categories: | 202 |
Online | |
---|---|
Active Users: | 105 |
Members: | 2 |
Guests: | 103 |
Bots: | 3462 |
Visits last 24h (live): | 2193 |
Visits last 24h (bots): | 26220 |